As we approach 2025, the landscape of WordPress development continues to evolve. In this piece, I intend to guide you (whether you’re a beginner or an advanced user) through the process of becoming better a developer by focusing on writing clean, object-oriented code and reducing reliance on plugins. Together, we’ll explore best practices, advanced techniques, and the benefits of a more streamlined, efficient approach to WordPress development.
As WordPress continues to power a significant portion of the web (an arguable 40%), the demand for skilled developers who can create efficient, scalable, and maintainable websites and applications is higher than ever. In 2025, the focus will shift towards writing custom, object-oriented code and moving away from the overreliance on plugins that has characterized much of WP development in the past (in my opinion, giving it an undeserved bad name).
The Case for Plugin-Free Development
Please don’t get me wrong!
Plugins have their place in the WP ecosystem. However, excessive use can lead to bloated, slow-loading websites and potential security vulnerabilities.
By writing custom code, developers can:
- Improve site performance and reduce load times
- Enhance security by minimizing third-party dependencies
- Create tailored functionality that precisely meets project requirements
- Maintain greater control over the codebase and its evolution
For beginners, this approach may seem daunting at first, but it offers an excellent opportunity to deepen your understanding of WordPress core and improve your PHP skills.
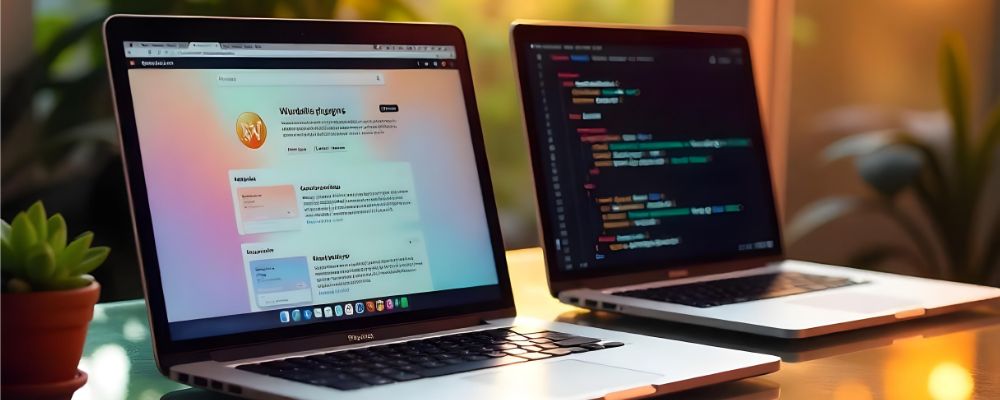
Embracing Object-Oriented Programming in WordPress
Object-Oriented Programming (OOP) is a powerful paradigm that can greatly enhance the maintainability and scalability of WP projects. Here’s how to get started:
For Beginners:
- Start by understanding basic OOP concepts: classes, objects, properties, and methods.
- Practice creating simple classes for common WordPress elements, such as custom post types or widgets.
For Advanced Users:
- Implement design patterns like Singleton, Factory, and Observer in your WordPress projects.
- Use namespaces and autoloading to organize your code more efficiently.
Example of a basic custom post type class:
class BusyBooks {
public function __construct() {
add_action('init', [$this, 'registerPostType']);
}
public function registerPostType() {
register_post_type('book', [
'labels' => [
'name' => 'Books',
'singular_name' => 'Book',
],
'public' => true,
'has_archive' => true,
'supports' => ['title', 'editor', 'thumbnail'],
]);
}
}
new BusyBooks();
The advantage of writing our own code for the BusyBooks post type over using a plugin is for us to have complete control over its implementation. We can easily customize it to fit our exact needs, whereas a plugin might offer more features than you need or lack specific functionality you require, which greatly affects performance and scalability.
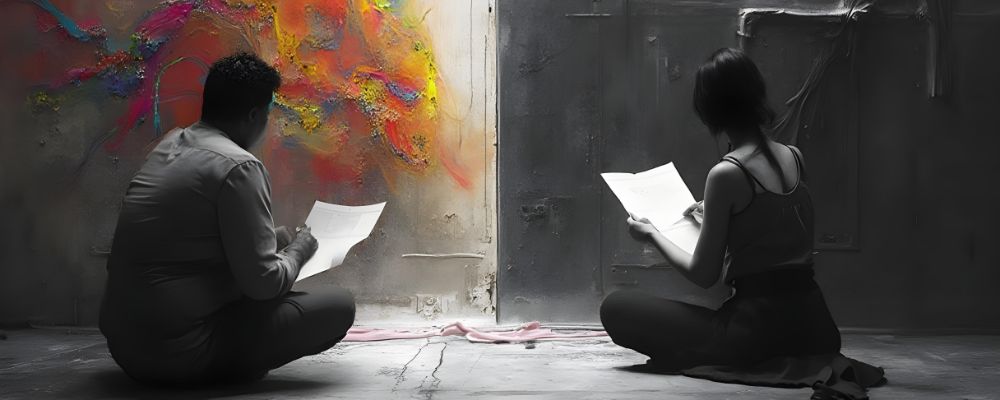
Advanced Techniques for Efficient WordPress Development
- Use Composer for Dependency Management: Integrate Composer into your WP projects to manage PHP dependencies and autoload classes.
- Implement the WordPress REST API: Create custom endpoints for your applications, enabling better integration with front-end frameworks.
- Utilize WordPress Hooks Effectively: Create modular, extensible code by leveraging action and filter hooks throughout your custom functionality.
- Embrace Modern PHP Features: Take advantage of PHP 7.4+ features like typed properties, arrow functions, and null coalescing assignment operators to write more robust code.
Best Practices for Code Organization and Readability
- Follow the WordPress Coding Standards to ensure consistency and readability.
- Use meaningful naming conventions for functions, classes, and variables.
- Implement a consistent file and directory structure for your themes and plugins.
- Write clear, concise comments and use DocBlocks for functions and classes.
Example of a well-structured plugin directory:
my-busy-plugin/
├── assets/
│ ├── css/
│ └── js/
├── includes/
│ ├── class-my-custom-plugin.php
│ ├── class-post-types.php
│ └── class-meta-boxes.php
├── templates/
│ └── admin/
├── tests/
├── my-custom-plugin.php
└── readme.txt
Performance Optimization Strategies
- Implement proper caching mechanisms, including object caching and page caching.
- Optimize database queries by using
$wpdb
prepared statements and indexing. - Minimize the use of external HTTP requests and implement request caching when necessary.
- Utilize WordPress transients for storing and retrieving temporary data.
Security Considerations in Custom Development
- Implement proper data sanitization and validation for all user inputs.
- Use WordPress nonces to protect against CSRF attacks.
- Follow the principle of least privilege when setting up user roles and capabilities.
- Regularly update your custom code to address potential vulnerabilities and stay compatible with the latest WordPress core updates.
Testing and Debugging Custom WordPress Code
- Implement unit testing using PHPUnit and the WP testing framework.
- Use debugging tools like Xdebug and the Query Monitor plugin during development.
- Set up continuous integration and automated testing workflows using tools like GitHub Actions.
Staying Updated with WordPress Core and Web Standards
- Follow the official WordPress developer blog and contribute to core when possible.
- Stay informed about upcoming changes to PHP and web standards.
- Participate in WordPress community events and conferences to network and learn from other developers.
Conclusion
Becoming a better WordPress developer in 2025 means embracing clean, efficient coding practices and reducing reliance on third-party plugins. By focusing on writing custom, object-oriented code, you’ll not only improve your skills but also create more performant, secure, and maintainable WordPress projects. Whether you’re a beginner or an advanced user, continuous learning and adaptation to evolving best practices will be key to your success in the WordPress ecosystem.