Pagination is an ordinal numbering of pages, which is usually located at the top or bottom of the site pages. In most cases, it is used for main pages and partitions. It often looks like this:
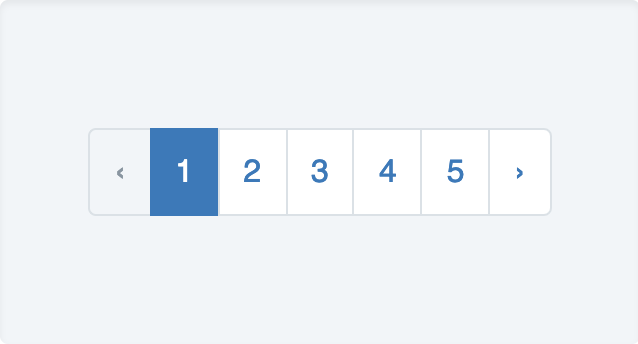
Lets look at the code:
function pagination($item_count, $limit, $cur_page, $link) { $page_count = ceil($item_count/$limit); $current_range = array(($cur_page-2 < 1 ? 1 : $cur_page-2), ($cur_page+2 > $page_count ? $page_count : $cur_page+2)); // First and Last pages $first_page = $cur_page > 3 ? '<a href="'.sprintf($link, '1').'">1</a>'.($cur_page < 5 ? ', ' : ' ... ') : null; $last_page = $cur_page < $page_count-2 ? ($cur_page > $page_count-4 ? ', ' : ' ... ').'<a href="'.sprintf($link, $page_count).'">'.$page_count.'</a>' : null; // Previous and next page $previous_page = $cur_page > 1 ? '<a href="'.sprintf($link, ($cur_page-1)).'">Previous</a> | ' : null; $next_page = $cur_page < $page_count ? ' | <a href="'.sprintf($link, ($cur_page+1)).'">Next</a>' : null; // Display pages that are in range for ($x=$current_range[0];$x <= $current_range[1]; ++$x) $pages[] = '<a href="'.sprintf($link, $x).'">'.($x == $cur_page ? '<strong>'.$x.'</strong>' : $x).'</a>'; if ($page_count > 1) return '<p class="pagination"><strong>Pages:</strong> '.$previous_page.$first_page.implode(', ', $pages).$last_page.$next_page.'</p>'; }
Example usage:
pagination($item_count = 45, $limit = 20, $cur_page = 1, $link= 'http://busystory.com/devs/%d.html');
Or a more simpler and cleaner version
pagination(45, 20, 1, 'http://busystory.com/devs/%d.html');
Output HTML:
<p class="pagination"> <strong>Pages:</strong> <a href="http://example.com/userlist/1.html"><strong>1</strong></a>, <a href="http://example.com/userlist/2.html">2</a>, <a href="http://example.com/userlist/3.html">3</a> | <a href="http://example.com/userlist/2.html">Next</a> </p>